Schedule integrations
Schedule integrations
In this article, you’ll learn how to use a schedule to invoke an integration at a future point in time.
What is scheduling?
Integrations can be scheduled and executed at a future time. There are two ways to do this:
-
Scheduling a single execution from within an experience
-
Scheduling a recurring execution running on a set schedule
|
Note
The upper limit for scheduling an integration is 30 days. Integration invocations beyond this limit will be rejected. |
Scheduling in an experience
The Integration API exposes 2 methods that facilitate scheduling, schedule
and cancel
:
module.exports = function variation (options) {
// Slack integration that posts messages to a specific channel
const integration = options.integration('slack-update')
// Payload is same as calling an integration directly
const payload = { message: 'Hello!' }
const options = {
key: {
foo: 'bar'
},
delay: 60 * 60 * 1000, // Amount of time to wait before executing
window: { // Window of time the integration is allowed to execute
day: 'monday-friday', // Range of days allowed
time: '09:00-17:00' // Time range allowed
}
}
integration.schedule(payload, options)
.catch((err) => {
options.log.error(`Could not schedule execution: ${err.message}`)
})
}
options.key: Object
The key object used to identify the scheduled execution.
options.delay: String | Integer
The delay before executing the action. Accepts an integer in milliseconds or a ms-compatible string representation.
|
Cannot be used together with options.time_ |
options.time: String | Integer
Accepts an integer representing the UNIX timestamp (milliseconds elapsed since 1st January 1970 00:00:00 UTC) or an ISO 8601^
formatted date string for example, 2018-08-29T14:17:46.175Z
.
|
Can’t be used together with options.delay |
options.window.day: String
Sets the days of the week when the integration can fire. If the integration is scheduled for a time outside this range, it will be delayed to next earliest time that satisfies this range.
-
"monday-friday"
for weekdays only -
default -
"sunday-saturday"
options.window.time: String
Sets the time of day when the integration can fire. If the integration is scheduled for a time outside this range, it will be delayed to next earliest time that satisfies this range.
-
"09:00-17:00"
for business hours only -
default -
"00:00-23:59"
options.window.timezoneOffset: Integer
Defines the timezone offset the defined window operates in. If the window object is provided without this value, it will automatically be populated from the visitor’s browser, this means that the window will be relevant to the user’s local time.
-
-60
for UTC+1
default: Local timezone of the visitor’s browser
options.cancelOnConversion: Boolean
If true, the scheduled execution will be automatically cancelled when a conversion is detected for the current visitor.
-
default -
false
Cancelling a scheduled execution
module.exports = function variation (options) {
// Slack integration that posts messages to a specific channel
const integration = options.integration('slack-update')
// Payload is same as calling an integration directly
const options = {
// The same unique key used when scheduling the execution
key: {
foo: 'bar'
}
}
integration.cancel(options)
.catch((err) => {
options.log.error(`Could not cancel scheduled execution: ${err.message}`)
})
}
options.key: Object
The key object used to identify the scheduled execution.
options.reason: String
The reason for cancellation.
Recurring schedule
Open your integration and select .
Now select at the bottom of the page.
This opens the Settings for your integration, where you can define a schedule:
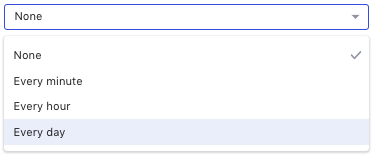