Capture a search event
Capture a search event
This article explains how to capture a search event and send the information to Coveo along with the required fields.
When to capture a search event
The search event is by far one of the most commonly logged commerce usage analytics events. Any time a ranked list of products is shown to a visitor, whether it be from submitting a search query, navigating to a listings page, or viewing a product recommendations carousel, a search event must be sent to the Coveo Platform to capture user intent and begin tracing the user journey. The event will be used to measure attribution from a search results page, a product listings page, or a recommendations component.
Headless controllers and Atomic components
Headless and Atomic components log search events under the hood on your behalf. You don’t need to manually log search events if you build your UI with these technologies.
The following sections provide guidance on the necessary configurations to ensure that search events are appropriately logged.
Initialization configuration
This section is dedicated to explaining the initial configuration of your components.
Specifically, we will discuss the importance of properly setting the searchHub
, pipeline
, and language
parameters.
Set the search hub
Depending on the type of interface the event originates from, there are naming conventions for the search hub value you need to follow. We recommend setting the search hub on Headless and Atomic to control how the queries are processed in the Coveo Administration Console (see Query routing).
When configured, the frameworks will automatically send the value in search events in the originLevel1
field.
You can either configure the search hub in the authentication method, or set it at interface level.
Language
In Coveo Machine Learning (ML) models, multilingual search and recommendations are supported, allowing you to deliver personalized and relevant content to users in different languages.
To ensure accurate and effective language-based results, it’s important to consider the language of the search query and align it with the language specified in the various usage analytics events.
Headless and Atomic automatically set the language of usage analytics events to the language of the search query.
The language is set to en
by default; however, if your use case serves content in multiple languages, we recommend specifying the language by setting the value of the locale
field when initializing your components.
The locale
field is formed by combining the language and region values with a hyphen, like en-US
, where en
represents the language and US
represents the region.
Query pipeline
When the interface sends a query to your Coveo organization, it goes through a specific Coveo query pipeline before reaching the index. This can be done by specifying a Search Hub and then using condition based routing in the Administration Console to target a specific pipeline.
A query pipeline uses its rules to transform that query and apply specific features following a specific order of execution. While it’s not necessary to specify the query pipeline, we recommend that you do, as it allows you to filter and segregate events when looking at usage analytics reports.
For Headless
The following code snippet explains how to set the searchHub
, and language
fields when initializing your Headless search engine.
Specify these values using the SearchConfigurationOptions
object, as in the following code sample:
import { buildSearchEngine, getOrganizationEndpoints } from '@coveo/headless';
export const headlessEngine = buildSearchEngine({
configuration: {
organizationId: '<ORGANIZATION_ID>',
accessToken: '<ACCESS_TOKEN>'
organizationEndpoints: getOrganizationEndpoints('<ORGANIZATION_ID>'),
search: {
searchHub: "<SEARCH_HUB>",
locale: "<LOCALE>",
},
},
});
For Atomic
The following code snippet explains how to set the searchHub
, and language
fields by setting these properties on the atomic-search-interface
component:
<body>
<atomic-search-interface
id="search"
search-hub="<SEARCH_HUB>"
language="<LANGUAGE>"
>
<atomic-search-box></atomic-search-box>
<!-- ... -->
</atomic-search-interface>
</body>
<body>
<atomic-search-interface id="search" language="<LANGUAGE>">
<atomic-search-box></atomic-search-box>
<!-- ... -->
</atomic-search-interface>
</body>
Origin levels
The originLevel
feature provides valuable information about the origin of usage analytic events, similar to the Search Hub.
Several fields are associated with the originLevel
feature:
-
originLevel1
(required): This field should match the value of the Search Hub. If you initialize Headless or Atomic with the SearchHub configuration, this field is automatically set. -
originLevel2
(recommended): This field lets you differentiate between different tabs within the search interface if multiple tabs are present. When using the Tab controller, Headless can automatically populate theoriginLevel2
field with the ID specified during the initialization of your controller.-
For the "Search" interface without any tabs, you can send the value "default".
-
For the "Listing" page, send the path name of the listing (for example, "my-products/shoes").
-
For "Recs", you can send the value "default".
-
-
originLevel3
(recommended): This field represents the URL of the page that redirects the browser to the search interface. It helps you analyze the effectiveness of various entry points leading to the search experience. It’s typically extracted fromdocument.referrer
.
During engine initialization, you can set the values for the originLevel
fields.
However, if you need to dynamically modify these values, you have the flexibility to modify the usage analytics data sent to Coveo.
You can do so by leveraging the analyticsClientMiddleware
property of an AnalyticsConfiguration
to hook into an analytics event payload before it’s sent to Coveo.
This can be done for both Headless and Atomic.
Custom data
Along with the regular fields added by Headless and Atomic, UA events must contain a customData
JSON with additional fields.
While the library adds some fields to this customData
JSON, there are other fields that have to be manually added using the Headless library:
|
Note
If you’re using the Atomic library, you’ll need to access Headless through Atomic. |
Sending user information
If your implementation includes an authentication mechanism, we highly recommend sending additional fields regarding the user performing the request.
To automatically send this user-oriented usage analytics information to the Coveo Platform, use a search token.
This involves generating search tokens while providing the name
, userDisplayName
, and userGroups
fields.
The generated search token is then used to send requests via Headless and Atomic.
|
Note
The |
To understand how to send user information if users are logged in, and you’re utilizing an API key for authentication, see User tracking and anonymizing UA data.
Capture a search event using Coveo UA library commands
We strongly recommend using either the Headless or Atomic libraries for capturing search events as these libraries are designed to facilitate the logging of such events by automatically including the appropriate metadata and simplifying the implementation process. However, if you choose not to use these libraries or want to track search events on a component that’s not powered by Coveo, you can still log search events manually using the Coveo Usage Analytics (UA) library.
The following is an example of the JavaScript code that uses the Coveo UA library to send a search event:
coveoua('send', 'search', {
'actionCause': 'searchboxSubmit',
'queryText': 'shoes',
'responseTime': 23,
'anonymous': true,
'originLevel1': 'MainSearch',
'originLevel2': 'default',
'originLevel3': 'http://localhost:3000/search',
'searchHub': 'MainSearch',
'searchQueryUid': '514970dd-7c76-429f-8e80-6ea2f957f303',
'language': 'en',
'trackingId': 'Sports',
});
Send the search event, specifying the various required fields. |
Search data payload requirements
The following table lists the fields sent along with the event and the solutions that leverage them. Search, Listings, and Recs columns refer to whether the ML that powers those solutions needs those data points. The Reporting column is for general reporting purposes that encompasses all solutions.
Field | Priority | Search | Listings | Recs | Reporting |
---|---|---|---|---|---|
Required |
|||||
Required |
|||||
Required |
|||||
Required |
|||||
Required |
|||||
Required |
|||||
Required |
|||||
Required |
|||||
Required |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
Recommended |
|||||
|
Recommended |
-
Defaults to English.
-
Refer to Extract the searchUid.
-
User specific fields which can be sent if the user is authenticated.
-
Field sent when the user interacts with a facet. Refer to Implement facets.
-
Field sent when the user interacts with Coveo ML query suggestions. Refer to Handle Coveo Machine Learning query suggestion selection.
-
Array containing items that represent active facets applied in the search. Refer to Implement facets.
Refer to Log search events for more information on the fields discussed above.
Search event validation
To validate that the search event was sent, use the developer tools for client-side request verification to inspect your visit to ensure that everything done using the UI is captured as a set of consistent and coherent events. This must occur after the implementation is applied.
Inspect the search event through the network browser and ensure that it satisfies the following:
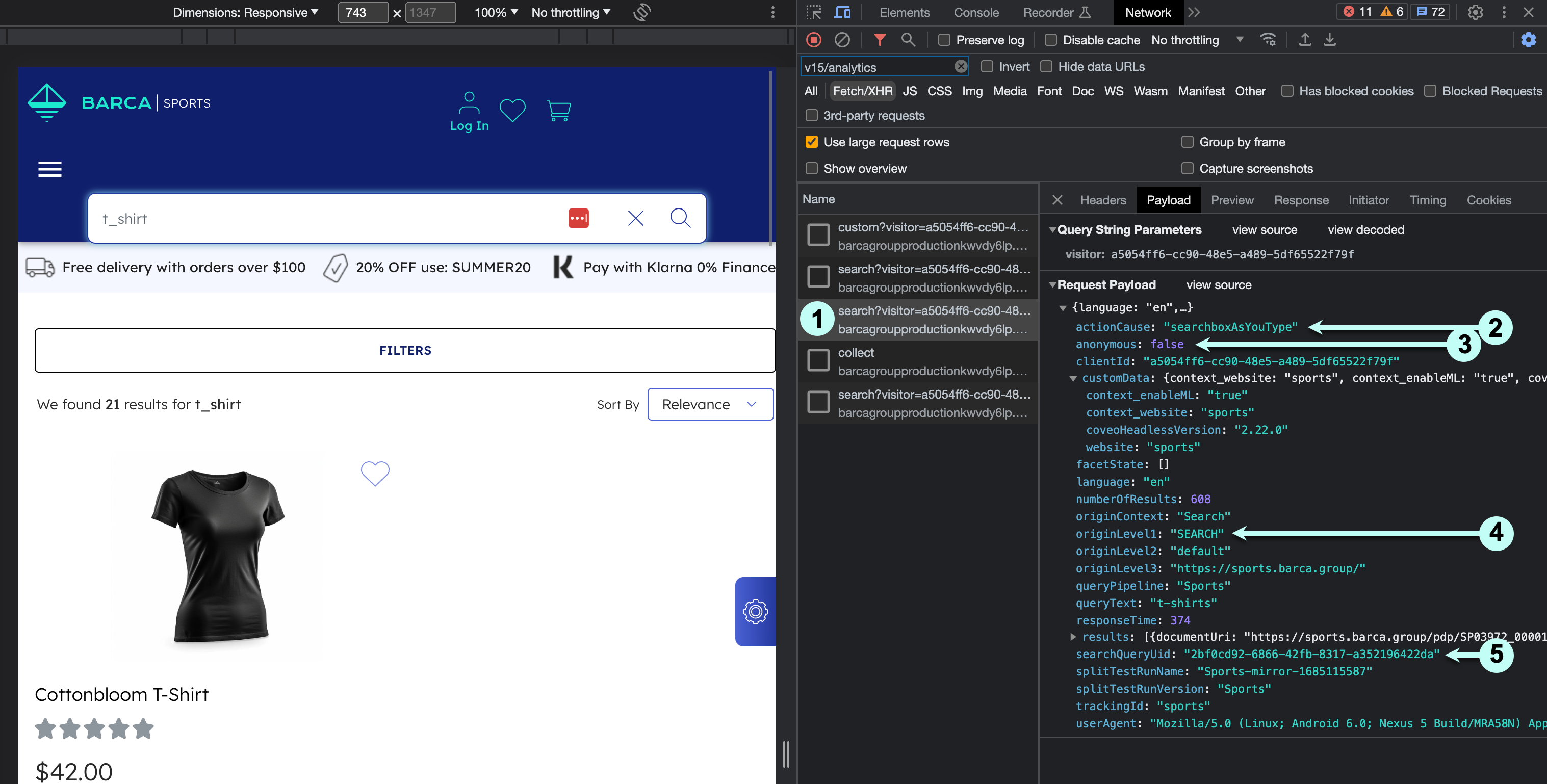
1 A search
event is sent whenever the user interacts with the search box or a facet.
2 The actionCause
parameter of the search event is attributed to the correct value.
Some common values include:
User action identifier | Description |
---|---|
Selecting or deselecting the facet that re-renders the search results page. |
|
Deselecting all facets, re-rendering search. |
|
When a user loads a search interface, for example, when reloading the search results page. |
|
When a search result originates from a query suggestion. |
|
A visitor views a recommendation. |
|
When a user submits search queries in the search box. |
|
A visitor clicks a navigation link and lands on the search results page. |
See the list of action causes for analytics purposes.
3 The anonymous
field is set to the correct value based on the implementation.
The default value is false
.
4 The value of the originLevel1
must contain a value to calculate the attributed value for each product discovery solution.
For example, search
for search, listing
for product listings, and rec
for product recommendations.
5 Ensure that the searchQueryUid
is unique for every search event.