Content recommendations
Content recommendations
The QuanticRecommendationInterface
and QuanticRecommendationList
components let you display Content Recommendations (CR) powered by Coveo Machine Learning (Coveo ML).
|
Note
CR models depend on view events and the user’s action history.
To display recommendations with Quantic, you must have configured a CR model and logged the required usage analytics events via the |
Implementation example
The following Lightning Web Component (LWC) example shows how you can implement a recommendation interface using the quantic-recommendation-interface
and quantic-recommendation-list
components.
HTML
<template>
<div class="search__container slds-p-around_medium"
onregisterresulttemplates={handleResultTemplateRegistration}>
<c-quantic-recommendation-interface
engine-id={engineId}
pipeline={pipeline}
search-hub={searchHub}>
<c-quantic-recommendation-list
engine-id={engineId}
number-of-recommendations={numberOfRecommendations}
recommendations-per-row={recommendationsPerRow}
label={label}
variant={variant}
fields-to-include={fieldsToInclude}
recommendation={recommendation}
>
</c-quantic-recommendation-list>
</c-quantic-recommendation-interface>
</div>
</template>
Optionally, register your own result templates to customize the appearance of your recommendation results, the same way you would in your main search interface. | |
The quantic-recommendation-interface component is the parent of all other Quantic components in a recommendation interface. |
|
quantic-recommendation-list displays recommendations by applying the options provided to it. |
JavaScript
import {LightningElement, api} from 'lwc';
// @ts-ignore
import caseTemplate from './resultTemplates/caseResultTemplate.html';
export default class ExampleQuanticRecommendationsList extends LightningElement {
@api engineId = 'quantic-recommendation-list-engine';
@api pipeline = 'mypipeline';
@api searchHub = 'mysearchhub';
@api numberOfRecommendations = 3;
@api recommendationsPerRow = 1;
@api label = "Recommended for you";
@api variant = "grid";
@api fieldsToInclude = "objecttype,sfstatus,sfcasestatus,sfcasenumber";
@api recommendation = "recommendation";
handleResultTemplateRegistration(event) {
event.stopPropagation();
const resultTemplatesManager = event.detail;
const isCase = CoveoHeadless.ResultTemplatesHelpers.fieldMustMatch(
'objecttype',
['Case']
);
resultTemplatesManager.registerTemplates(
{
content: caseTemplate,
conditions: [isCase],
fields: ['sfstatus', 'sfcasestatus', 'sfcasenumber'],
}
);
}
}
Import your custom result templates, if any. | |
The engineId is the unique identifier of the Headless engine that powers the recommendation interface.
We recommend that you set a different engine from the one powering your search interface. |
|
The searchHub and pipeline properties are required to correctly make requests to the Coveo Search API and usage analytics Write API.
Although we recommend that you enforce these properties through the search token, you should still set them in your component to ensure that it works as expected. |
|
The fieldsToInclude property is a comma-separated list of fields to include in the recommendation results.
These fields are used to build the result templates and to display the recommendations. |
|
The recommendation identifier used by Coveo ML to scope the recommendation interface. | |
Define result templates the same way you would in your main search interface. |
XML
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>57.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
<target>lightning__AppPage</target>
<target>lightning__HomePage</target>
<target>lightningCommunity__Page</target>
<target>lightningCommunity__Default</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordPage, lightning__AppPage, lightning__HomePage, lightningCommunity__Page, lightningCommunity__Default">
<property name="numberOfRecommendations" type="Integer" default="3" label="Enter the number of recommendations in total"/>
<property name="recommendationsPerRow" type="Integer" default="1" label="Enter the number of recommendations per row"/>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Set the targets of your LWC. | |
Optionally, expose certain parameters through the Salesforce Experience Builder. |
Generate search tokens for your component
We recommend that you create a search token provider dedicated to your recommendation component. Enforce the search hub through this provider to securely ensure that the component always has the required context.
-
If you’ve installed and configured Coveo for Salesforce v4 or a later package in your Salesforce organization, it’s typically enough to copy the
CoveoTokenProvider
Apex class, along with itsmeta.xml
file, into your Salesforce DX project. This class can automatically fetch your Salesforce users' information to generate search tokens for them. This is the best option for most use cases.If you haven’t installed Coveo for Salesforce v4 or a later package, or if the
CoveoTokenProvider
Apex class doesn’t fit your needs, you’ll have to implement your own search token provider.You can find an example in the Quantic project repository.
-
Once you’ve copied or created your search token provider Apex class, you need to modify the Headless engine configuration used by the recommendation controller so that it calls your Apex class. You can modify the
RecommendationsController
file atforce-app/quantic/classes
. Locate the following line:return SampleTokenProvider.getHeadlessConfiguration();
Modify it so that it leverages your search token provider instead:
return YOUR_RECOMMENDATION_TOKEN_PROVIDER.getHeadlessConfiguration();
where you replace
YOUR_RECOMMENDATION_TOKEN_PROVIDER
with the name of the search token provider Apex class that you created for your recommendation interface. -
After you deploy your project, your changes will be effective in your Salesforce organization.
Experiment with different variants and dimensions
The quantic-recommendation-list
component supports different variants to display recommendations.
Experiment with them to judge which one best fits your use case.
grid
The grid
variant displays recommendations in a grid layout, with the numberOfRecommendations
and number of recommendationsPerRow
of your choice.
-
numberOfRecommendations
set to3
andrecommendationsPerRow
set to1
-
numberOfRecommendations
set to9
andrecommendationsPerRow
set to3
carousel
The carousel
variant displays recommendations in a carousel layout, with the numberOfRecommendations
and number of recommendationsPerRow
of your choice.
numberOfRecommendations
set to 9
and recommendationsPerRow
set to 3
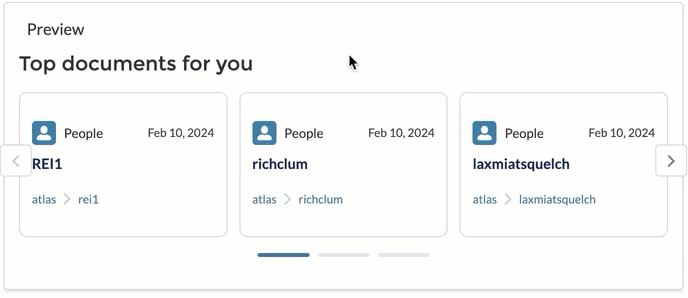