Implementation guide
Implementation guide
Regardless of the approach you choose to build your Coveo Platform-powered search interface, follow the implementation guidelines in this article.
By default, the search interfaces you create will display items from all your Coveo organization sources in the search results.
The following diagram gives a high-level view of the server-side tasks you need to code to build a functional search interface. The diagram also shows the client-side event flow before the search page is ready for use. More details about specific pieces of the implementation are provided further below.
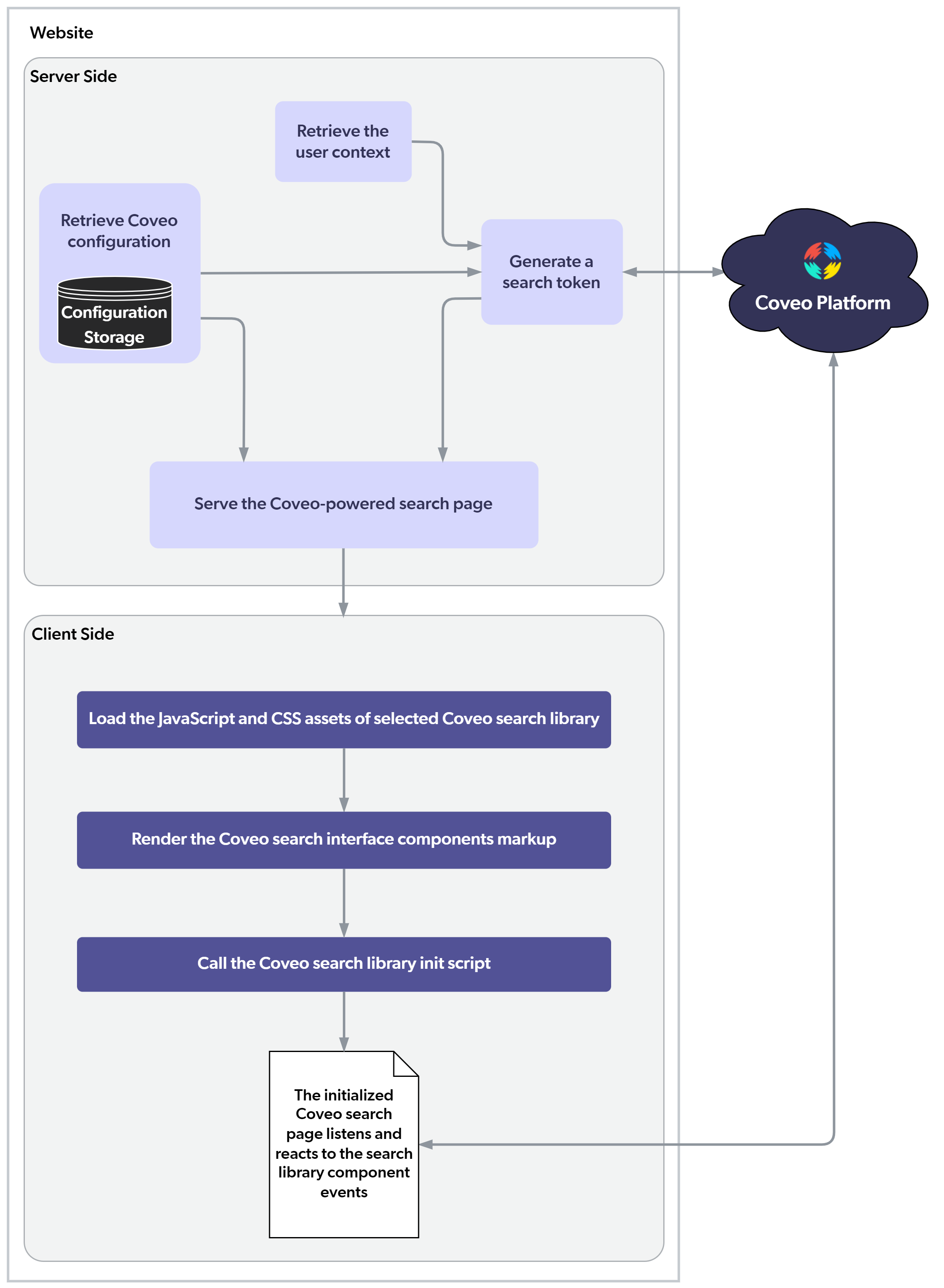
Retrieve the Coveo-related configuration
To link a search interface to your Coveo organization, configuration is required. You want to store the configuration parameters in your CMS. Minimally, these should include the following:
The platform endpoint URL
Coveo exposes organization-specific endpoints such as <ORG_ID>.org.coveo.com
to perform queries, where <ORG_ID>
is the unique identifier of your organization.
Under the hood, Coveo will redirect you based on your primary deployment region:
-
Canada: platform-ca.cloud.coveo.com
-
Australia: platform-au.cloud.coveo.com
-
Europe: platform-eu.cloud.coveo.com
Store the <ORG_ID>.org.coveo.com
endpoint associated with your organization in your CMS.
The Coveo organization ID
Calls to the Coveo APIs require that you specify your organization ID. This information may be found in the Coveo Administration Console, on the Settings (platform-ca | platform-eu | platform-au) page, under Organization > Details.
The search hub
The search hub is an important piece of information that your search interface should send to the Search API in its queries. When creating your Search API key, you will limit the scope of the key to this search hub. Learn more about the search hub.
|
If you’re using the Atomic library, you will set the search hub value in your search interface using the |
The Search API key
On the API Keys (platform-ca | platform-eu | platform-au) page, create an API key and, in the Privileges tab, grant the following privileges:
Service | Domain | Access level |
---|---|---|
Analytics |
Analytics data |
Push |
Search |
Impersonate |
Allowed |
|
To follow leading security practices, you need to limit the scope of your Search API key to a given search hub. The Add key button is inactive until a search hub is selected. ![]() |
The Search API key will be used to generate a search token.
|
Never expose a Search API key in client-side code. |
Retrieve the user context
You need to retrieve the user context to generate a search token for the corresponding security identity in your Coveo organization.
Generate a search token
Your server-side code will use the Search API key from your configuration storage and the security identity to request a search token for that user (see Sample usage workflow).
To get the full URL of your search token POST
call, append /rest/search/v2/token
to your platform endpoint URL.
Regardless of your data residency region, the full URL of your search token POST
call is https://<ORG_ID>.org.coveo.com/rest/search/v2/token
where <ORG_ID>
is replaced with your unique organization ID.
Your Coveo search library client-side code will use the search token to authenticate requests to the Coveo Search API.
|
Never expose a Search API key in client-side code. The generated search token can be exposed as it’s scoped to the current user context. |
|
Search token authentication is only one of several methods you can use to authenticate calls to the Coveo REST API. |
Serve the Coveo-powered search page
You need to write your search page HTML and JavaScript sections, following the reference documentation of the search interface library you chose to use.
|
The Coveo Atomic library is the recommended approach to building your Coveo-powered search pages in websites. See the following resources to build an Atomic search page:
|
You have decided to use Coveo Atomic as your search interface library. You can use your stored and generated constants as follows:
Search Token and Coveo organization ID: Use these values in the searchInterface initialize
method, for the accessToken
, organizationId
, and organizationEndpoints
arguments, as follows:
<script>
(async () => {
await customElements.whenDefined("atomic-search-interface");
const searchInterface = document.querySelector("#search");
await searchInterface.initialize({
accessToken: "eyJhbGciOiJIUzI1NiJ9eyM2OCI6dHJ1ZSwib3JnYW5pemF0aW9uIjoibWR0ZXN0MjIyc2VySWRzIm92a...",
organizationId: "mtest211p9s2x",
organizationEndpoints: await searchInterface.getOrganizationEndpoints('mtest211p9s2x')
});
searchInterface.executeFirstSearch();
})();
</script>
For a HIPAA organization, pass hipaa as an argument to getOrganizationEndpoints , as follows:
|
Search hub: Use this value for the atomic-search-interface
component searchHub
property.
When the search interface is ready for use
Once initialized, the search interface listens and reacts to events on its components. For example, if you type a query in the search box and then click the submit button, the search interface component will call the Coveo Search API using the search token. The Search API response will be a list of results.
By default, the atomic-search-interface
component automatically sends UA events to the region-specific analytics endpoint along with every search call.
|
With the JavaScript Search Framework, you need to add the |
Be aware that the atomic-search-interface
component reflectStateInUrl
property default value is true
.
This setting appends the basic query expression (q) to your search page URLs as a URI fragment and may conflict with web server configurations.