Pass custom parameters to computed fields
Pass custom parameters to computed fields
Sometimes, you may want to pass custom parameters to a computed field through the configuration.
For example, following the Create a computed date field tutorial, you may want to control when the archivedate
field is created on items.
This tutorial shows how to configure the number of days before an item is archived.
Prerequisite
You have already completed the Create a computed date field tutorial.
Step 1: Update the Coveo.Custom project
-
Using Visual Studio, open the ArchiveDateField class.
-
Add a constructor to accept additional XML configuration.
private readonly XmlNode m_Configuration; public ArchiveDateField(XmlNode p_Configuration) { m_Configuration = p_Configuration; }
-
Add a method to parse the configuration XML attributes and return their value.
protected string GetConfigurationValue(string p_ConfigurationKey) { string configurationValue = null; if (m_Configuration != null && m_Configuration.Attributes != null) { XmlAttribute configurationAttribute = m_Configuration.Attributes[p_ConfigurationKey]; if (configurationAttribute != null) { configurationValue = configurationAttribute.Value; } } return configurationValue; }
-
Add a property to retrieve the configuration value formatted as an integer.
protected int DaysBeforeArchiving { get { int daysCount; if (!Int32.TryParse(GetConfigurationValue("daysBeforeArchiving"), out daysCount)) { daysCount = 365; // One year by default } return daysCount; } }
-
Change the
ComputeFieldValue
method to use theDaysBeforeArchiving
property./// <inheritdoc /> public object ComputeFieldValue(IIndexable p_Indexable) { SitecoreIndexableItem indexableItem = p_Indexable as SitecoreIndexableItem; if (indexableItem != null) { IIndexableDataField dateField = indexableItem.GetFieldByName(SITECORE_CREATED_FIELD); if (dateField != null) { DateTime itemCreationDate = DateUtil.ParseDateTime(dateField.Value.ToString(), DateTime.MaxValue); return itemCreationDate.AddDays(DaysBeforeArchiving).ToString(COVEO_INDEX_DATE_FORMAT); } } return null; }
-
Compile your project.
-
Copy the resulting assembly (
Coveo.Custom.dll
) to thebin
folder of your Sitecore instance.
Step 2: Update the computed field configuration
Open your Coveo.SearchProvider.Custom.config
file and add the daysBeforeArchiving
parameter as an XML attribute.
<field fieldName="archivedate" daysBeforeArchiving="30">Coveo.Custom.ArchiveDateField, Coveo.Custom</field>
Step 3: Rebuild your indexes
Step 4: Validate the computed field values
Validate the archivedate
field values on your items using the Content Browser (platform-ca | platform-eu | platform-au).
There should now be a difference of 30 days between the created
and archivedate
fields values.
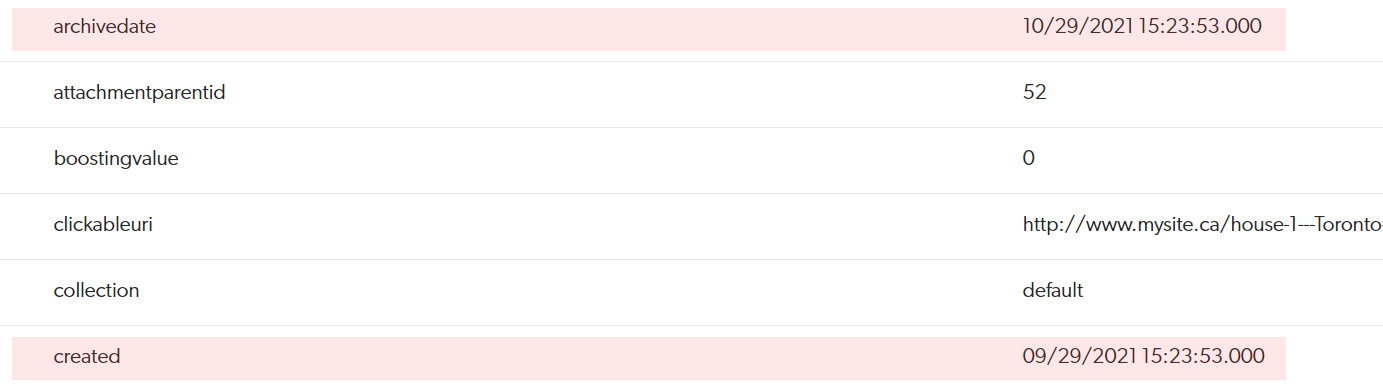
|
When running computed field code, check your logs for computed field errors indicating items aren’t being indexed. Implement proper exception handling to avoid these issues. For example, you might want to stop the process or set a fallback value for a computed field when suitable. |