Create a computed date field
Create a computed date field
Suppose you want to create some kind of archiving system in Sitecore by adding a computed field.
For example, you would like to add an archivedate
whose value would be one year after the item creation date (that is, created
field in the example below).
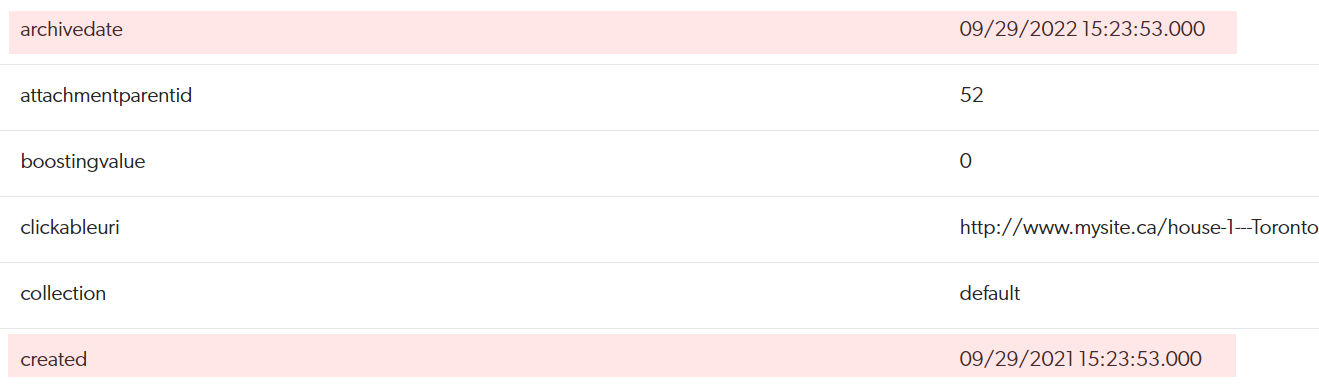
This article provides the steps to achieve this.
|
The Coveo Platform system date field and Coveo for Sitecore date field formats are incompatible. You can’t use a mapping to populate a Coveo Platform system date field with a Coveo for Sitecore computed date field. |
|
Note
If necessary, see Implement and configure a computed field in your index for more details on each step. |
Step 1: Create the required C# project
-
Create a new C# project named
Coveo.Custom
that references theSitecore.ContentSearch
andSitecore.Kernel
assemblies. -
Create a new class named
ArchiveDateField
in theCoveo.Custom
namespace with the following code.using System; using Sitecore; using Sitecore.ContentSearch; using Sitecore.ContentSearch.ComputedFields; namespace Coveo.Custom { public class ArchiveDateField : IComputedIndexField { // The 'Z' at the end of the format string tells Coveo // that the date value represents Universal time. private const string COVEO_INDEX_DATE_FORMAT = "yyyy/MM/dd@HH:mm:ssZ"; private const string SITECORE_CREATED_FIELD = "__Created"; /// <inheritdoc /> public string FieldName { get; set; } /// <inheritdoc /> public string ReturnType { get { return "datetime"; } set { } } /// <inheritdoc /> public object ComputeFieldValue(IIndexable p_Indexable) { SitecoreIndexableItem indexableItem = p_Indexable as SitecoreIndexableItem; if (indexableItem != null) { IIndexableDataField dateField = indexableItem.GetFieldByName(SITECORE_CREATED_FIELD); if (dateField != null) { DateTime itemCreationDate = DateUtil.ParseDateTime(dateField.Value.ToString(), DateTime.MaxValue); // Ensure that the date uses universal time if (itemCreationDate.Kind != DateTimeKind.Utc) { itemCreationDate = itemCreationDate.ToUniversalTime(); } return itemCreationDate.AddYears(1).ToString(COVEO_INDEX_DATE_FORMAT); } } return null; } } }
To create a computed date field, the
ReturnType
property must return thedatetime
string and theComputeFieldValue
method must return the date formatted asyyyy/MM/dd@HH:mm:ssZ
. -
Compile your project.
-
Copy the resulting assembly (
Coveo.Custom.dll
) to thebin
folder of your Sitecore instance.
Step 2: Configure the computed field
-
In
Coveo.SearchProvider.Custom.config
, add the following element as a child of the<fields hint="raw:AddComputedIndexField">
element.<field fieldName="archivedate">Coveo.Custom.ArchiveDateField, Coveo.Custom</field>
-
In
Coveo.SearchProvider.Custom.config
, add the following element as a child of the<fieldNames hint="raw:AddFieldByFieldName>
element.<fieldType fieldName="archivedate" settingType="Coveo.Framework.Configuration.FieldConfiguration, Coveo.Framework" returnType="System.DateTime" />
Step 3: Rebuild your indexes
Step 4: Validate the computed field has been added
Validate that your new field has been added on your items using the Content Browser (platform-ca | platform-eu | platform-au). Its value should be one year after the item creation date.
|
When running computed field code, check your logs for computed field errors indicating items aren’t being indexed. Implement proper exception handling to avoid these issues. For example, you might want to stop the process or set a fallback value for a computed field when suitable. |