Integrate a search page into your commerce solution
Integrate a search page into your commerce solution
This article serves as an introduction and high-level guide to creating a search interface with the Coveo Atomic library.
|
This section provides information on how to implement search interfaces that interact with the Coveo Search API, which is no longer recommended for Coveo for Commerce implementations. New Coveo for Commerce implementations should use the Coveo for Commerce API, which provides a more efficient and flexible way to interact with the Coveo Platform. See Build commerce interfaces for documentation on how to build commerce interfaces using the Coveo for Commerce API. |
Example code
You can quickly build a minimal search interface using just a few dozen lines of HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<!-- ... -->
<script
type="module"
src="https://static.cloud.coveo.com/atomic/v2/atomic.esm.js">
</script>
<link
rel="stylesheet"
href="https://static.cloud.coveo.com/atomic/v2/themes/coveo.css"/>
<script>
(async () => {
await customElements.whenDefined('atomic-search-interface');
const searchInterface = document.querySelector('#search');
await searchInterface.initialize({
accessToken:'<ACCESS_TOKEN>',
// Replace with an API key or search token that grants the privileges to execute queries and push usage analytics data.
organizationId: '<ORGANIZATION_ID>',
// Replace with your actual organization ID.
});
searchInterface.executeFirstSearch();
})();
</script>
<!-- ... -->
</head>
<body>
<atomic-search-interface search-hub="<SEARCH_HUB>">
<atomic-search-layout mobile-breakpoint="800px">
<atomic-layout-section section="search">
<atomic-search-box></atomic-search-box>
</atomic-layout-section>
<atomic-layout-section section="facets">
<atomic-facet-manager>
<atomic-facet field="cat_gender" label="Gender"></atomic-facet>
<atomic-facet field="store_name" label="Store"></atomic-facet>
<!-- ... -->
</atomic-facet-manager>
</atomic-layout-section>
<atomic-layout-section section="main">
<!-- ... -->
<atomic-layout-section section="results">
<atomic-result-list image-size="large" display="grid">
<atomic-result-template>
.
.
.
</atomic-result-template>
</atomic-result-list>
</atomic-layout-section>
<atomic-layout-section section="pagination" style="flex-direction: column">
<atomic-results-per-page choices-displayed="3,5,8,10"></atomic-results-per-page>
<atomic-load-more-results></atomic-load-more-results>
</atomic-layout-section>
</atomic-layout-section>
</atomic-search-layout>
</atomic-search-interface>
</body>
</html>
The rest of this article dissects and analyzes the various sections of the above code sample.
Including the Atomic library resources
To use the Atomic library, link the following two files in the <head>
of the page (see install the Atomic library):
<script
type="module"
src="https://static.cloud.coveo.com/atomic/v2/atomic.esm.js">
</script>
<link
rel="stylesheet"
href="https://static.cloud.coveo.com/atomic/v2/themes/coveo.css"/> 
The main Atomic script. | |
The default Atomic stylesheet. While this import is optional, if you don’t import it you’ll need to define its variables yourself. |
Initializing the Atomic search interface
Before you can begin searching content, you must connect your search page to your Coveo organization.
Do so within the <head>
tag of the search page.
<head>
<!-- ... -->
<script>
(async () => {
await customElements.whenDefined('atomic-search-interface');
const searchInterface = document.querySelector('#search');
await searchInterface.initialize({
accessToken: '<ACCESS_TOKEN>',
organizationId: '<ORGANIZATION_ID>',
renewAccessToken: <CALLBACK>,
});
searchInterface.executeFirstSearch();
})();
</script>
<!-- ... -->
</head>
<ACCESS_TOKEN> (string) is a search token or an API key that grants the Allowed access level on the Execute Queries domain and the Push access level on the Analytics Data domain in the target organization.
|
|||
<ORGANIZATION_ID> (string) is the unique identifier of your Coveo organization (for example, mycoveoorganizationa1b23c ). |
|||
<CALLBACK> (function) returns a new access token, usually by fetching it from a backend service that can generate search tokens.
The engine will automatically run this function when the current access token expires (that is, when the engine detects a 419 Authentication Timeout HTTP code).
|
Building your search interface
A Coveo Atomic search interface builds search pages by attaching components to target HTML elements. These components add functionality to your search interface. Some of the important components are highlighted in the example below.
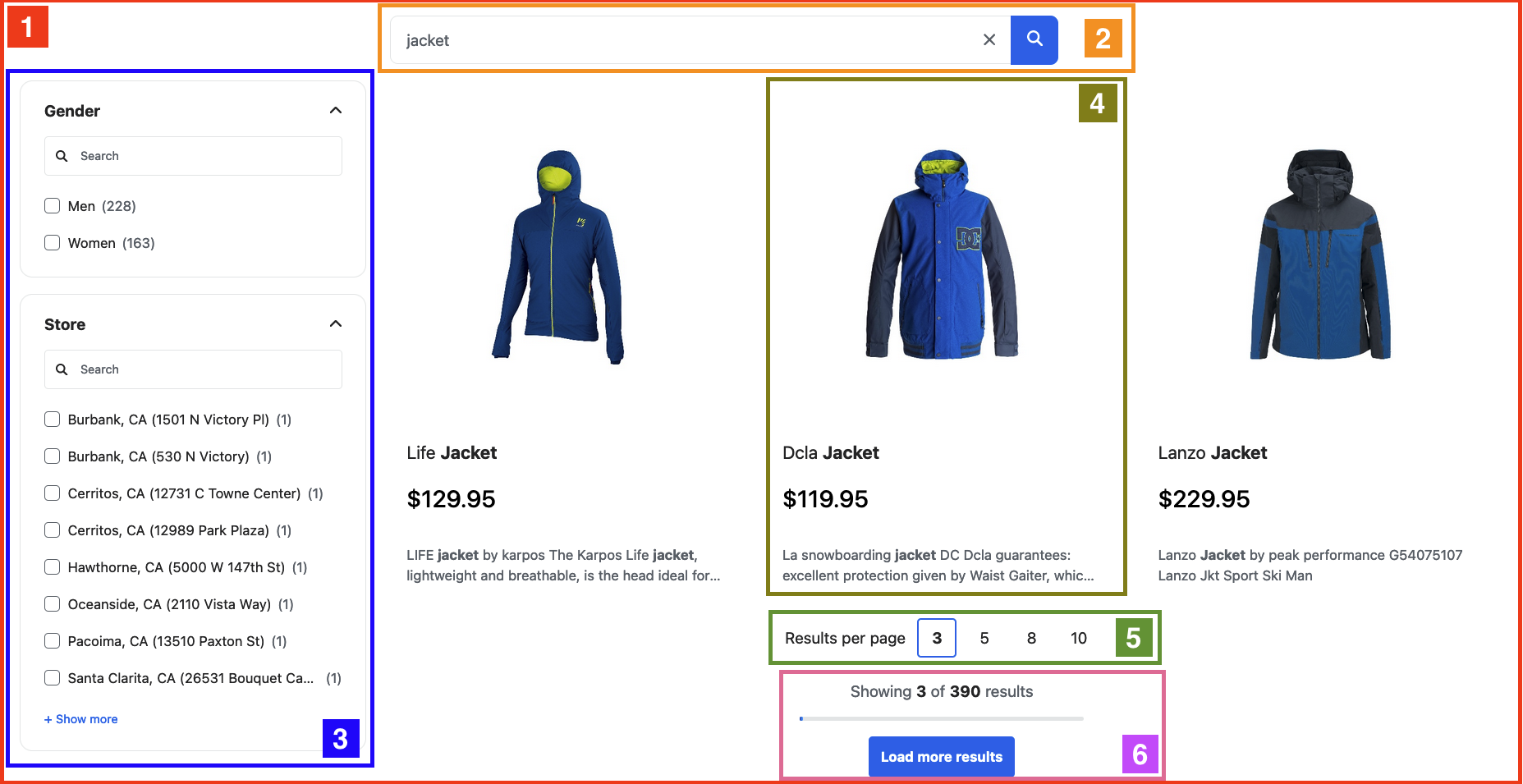
<body>
<atomic-search-interface search-hub="<SEARCH_HUB>">
<atomic-search-layout mobile-breakpoint="800px">
<atomic-layout-section section="search">
<atomic-search-box></atomic-search-box>
</atomic-layout-section>
<atomic-layout-section section="facets">
<atomic-facet-manager>
<atomic-facet field="cat_gender" label="Gender"></atomic-facet>
<atomic-facet field="store_name" label="Store"></atomic-facet>
<!-- ... -->
</atomic-facet-manager>
</atomic-layout-section>
<atomic-layout-section section="main">
<!-- ... -->
<atomic-layout-section section="results">
<atomic-result-list image-size="large" display="grid">
<atomic-result-template>
.
.
.
</atomic-result-template>
</atomic-result-list>
</atomic-layout-section>
<atomic-layout-section section="pagination" style="flex-direction: column">
<atomic-results-per-page choices-displayed="3,5,8,10"></atomic-results-per-page>
<atomic-load-more-results></atomic-load-more-results>
</atomic-layout-section>
</atomic-layout-section>
</atomic-search-layout>
</atomic-search-interface>
</body>
Some essential components include:
Atomic component | Description |
---|---|
The parent of all other atomic components in the search page.
Notice that this is where you would define a |
|
Helps organize elements on the search page.
Has the
|
|
Allows the identification of various sections of an Atomic page. Acts as a container
|
|
Helps reorder facets. Acts as a container for facet components.
|
|
5. atomic-facet |
Displays a list of values for a specific field occurring in the results
|
Responsible for displaying query results.
|
There’s another essential functionality which isn’t visible in the search interface example provided above: Atomic Usage Analytics. Atomic records usage analytics events performed in the search interface to feed Coveo Machine Learning (Coveo ML) models and to create UA reports.
For more details, to customize or send your own UA events, see Atomic Usage Analytics.
What’s next?
You now have a general understanding of how to configure a Coveo-powered search interface using the Atomic library.
You may want to follow up by going through the Coveo Atomic tutorial to better familiarize yourself with its concepts, components, functions, and methods.
You may also want to create a standalone search box to provide relevant search throughout your ecommerce solution or website.
For more examples, have a look at the interactive ones below: