Updating the Printable and Clickable URIs
Updating the Printable and Clickable URIs
The following pre-conversion indexing pipeline extension (IPE) script sample updates the item printable and clickable URIs.
|
Note
The following script sample assumes that your extension is already applied to a source. If you haven’t yet applied the extension to a source, refer to this article first. |
from functools import reduce
def get_flattened_meta():
flattened = dict()
for metadata in document.get_meta_data():
for metadata_name, metadata_values in metadata.values.items():
lowercase_metadata_name = metadata_name.lower()
if len(metadata_values) == 1:
flattened[lowercase_metadata_name] = metadata_values[0]
elif len(metadata_values) > 1:
flattened[lowercase_metadata_name] = ";".join([str(value) for value in metadata_values])
return flattened
def search_and_replace_from_list(string_replacements, original_string):
return reduce(lambda current_value, key_name: str(current_value).replace(key_name, string_replacements[key_name]),
string_replacements,
original_string or '')
uri_replacements = {
"value_to_replace:": "replacement_value",
# ...
}
meta_data = get_flattened_meta()
# Update the item printable URI (the URI the user sees on a search result).
document.add_meta_data({'printableuri':search_and_replace_from_list(uri_replacements, meta_data['printableuri']
if 'printableuri' in meta_data else '')})
# Update the item clickable URI (the URI that's opened when a user clicks a search result title).
document.add_meta_data({'clickableuri':search_and_replace_from_list(uri_replacements, meta_data['clickableuri']
if 'clickableuri' in meta_data else '')})
Example
You have a Web source containing content about your favorite movies. When users click one of those movies, they’re currently redirected as per the URIs extracted by the crawlers. You want to overwrite those URIs to instead redirect users to your personal web site.
Sample Movie Metadata
{
// ...
"title": "Ex Machina",
"data": "Caleb Smith, a young programmer, gets a chance to become a part of a strange scientific experiment where he is expected to assess artificial intelligence by interacting with a female robot.",
"author": "Alex Garland",
"theaters": ["The Universe Cinema", "The Prodigy Theatre", "The Ornate Opera House"],
"clickableuri": "http://ilovemovies.com/movies-details/ex-machina.html",
"printableuri": "http://ilovemovies.com/movies-details/ex-machina.html"
// ...
}
The new item appears in the Content Browser (platform-ca | platform-eu | platform-au) as such:
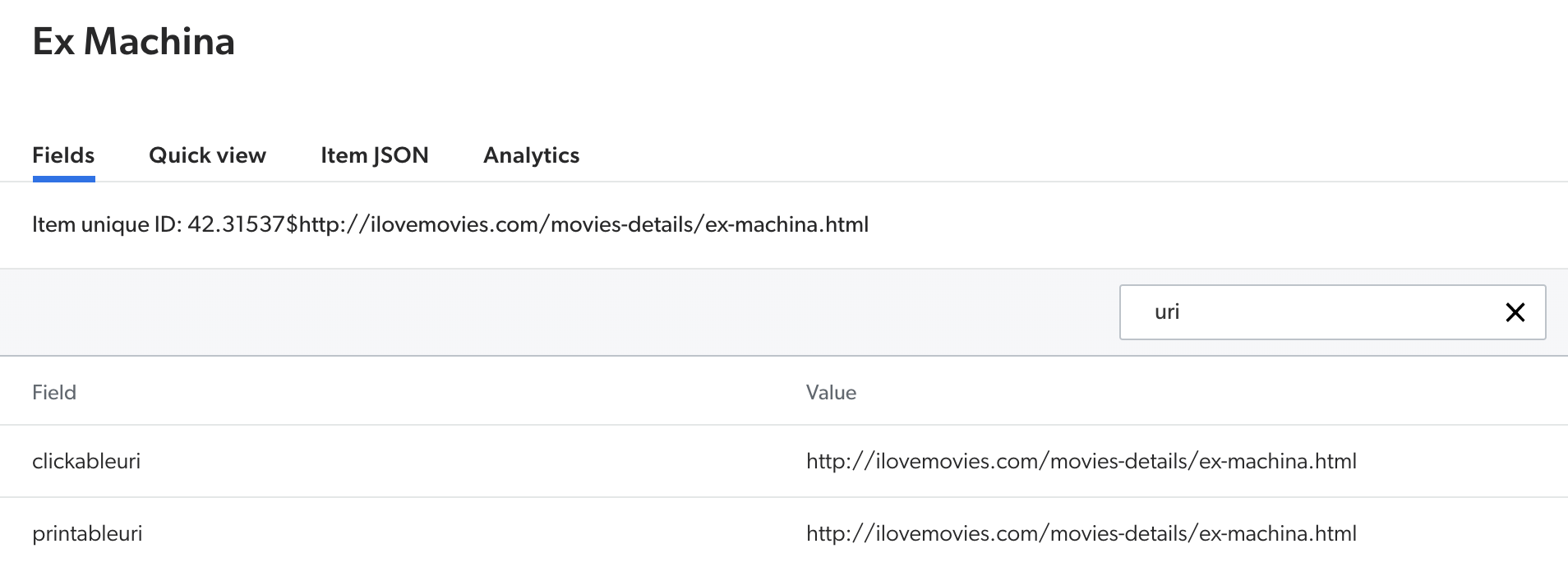
You configure the pre-conversion extension script with the following URIs:
uri_replacements = {
"http:": "https:",
"ilovemovies.": "moviemania.",
"/movies-details": "/movies"
}
You rebuild your source, and as a result the new item appears in the Content Browser as such:
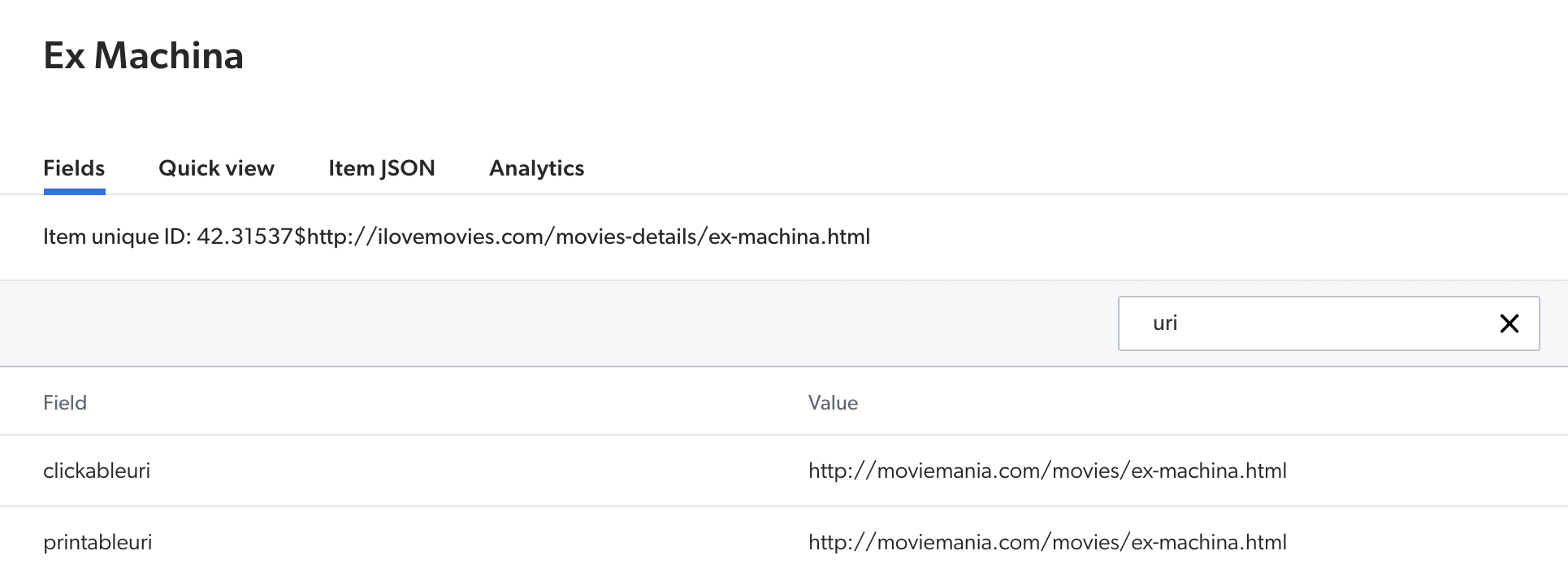